forked from luck/tmp_suning_uos_patched
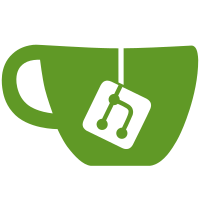
Writeback connectors represent writeback engines which can write the CRTC output to a memory framebuffer. Add a writeback connector type and related support functions. Drivers should initialize a writeback connector with drm_writeback_connector_init() which takes care of setting up all the writeback-specific details on top of the normal functionality of drm_connector_init(). Writeback connectors have a WRITEBACK_FB_ID property, used to set the output framebuffer, and a WRITEBACK_PIXEL_FORMATS blob used to expose the supported writeback formats to userspace. When a framebuffer is attached to a writeback connector with the WRITEBACK_FB_ID property, it is used only once (for the commit in which it was included), and userspace can never read back the value of WRITEBACK_FB_ID. WRITEBACK_FB_ID can only be set if the connector is attached to a CRTC. Changes since v1: - Added drm_writeback.c + documentation - Added helper to initialize writeback connector in one go - Added core checks - Squashed into a single commit - Dropped the client cap - Writeback framebuffers are no longer persistent Changes since v2: Daniel Vetter: - Subclass drm_connector to drm_writeback_connector - Relax check to allow CRTC to be set without an FB - Add some writeback_ prefixes - Drop PIXEL_FORMATS_SIZE property, as it was unnecessary Gustavo Padovan: - Add drm_writeback_job to handle writeback signalling centrally Changes since v3: - Rebased - Rename PIXEL_FORMATS -> WRITEBACK_PIXEL_FORMATS Chances since v4: - Embed a drm_encoder inside the drm_writeback_connector to reduce the amount of boilerplate code required from the drivers that are using it. Changes since v5: - Added Rob Clark's atomic_commit() vfunc to connector helper funcs, so that writeback jobs are committed from atomic helpers - Updated create_writeback_properties() signature to return an error code rather than a boolean false for failure. - Free writeback job with the connector state rather than when doing the cleanup_work() Changes since v7: - fix extraneous use of out_fence that is only introduced in a subsequent patch. Changes since v8: - whitespace changes pull from subsequent patch Changes since v9: - Revert the v6 changes that free the writeback job in the connector state cleanup and return to doing it in the cleanup_work() function Signed-off-by: Brian Starkey <brian.starkey@arm.com> [rebased and fixed conflicts] Signed-off-by: Mihail Atanassov <mihail.atanassov@arm.com> [rebased and added atomic_commit() vfunc for writeback jobs] Signed-off-by: Rob Clark <robdclark@gmail.com> Signed-off-by: Liviu Dudau <liviu.dudau@arm.com> Reviewed-by: Eric Anholt <eric@anholt.net> Link: https://patchwork.freedesktop.org/patch/229037/
92 lines
2.5 KiB
C
92 lines
2.5 KiB
C
/* SPDX-License-Identifier: GPL-2.0 */
|
|
/*
|
|
* (C) COPYRIGHT 2016 ARM Limited. All rights reserved.
|
|
* Author: Brian Starkey <brian.starkey@arm.com>
|
|
*
|
|
* This program is free software and is provided to you under the terms of the
|
|
* GNU General Public License version 2 as published by the Free Software
|
|
* Foundation, and any use by you of this program is subject to the terms
|
|
* of such GNU licence.
|
|
*/
|
|
|
|
#ifndef __DRM_WRITEBACK_H__
|
|
#define __DRM_WRITEBACK_H__
|
|
#include <drm/drm_connector.h>
|
|
#include <drm/drm_encoder.h>
|
|
#include <linux/workqueue.h>
|
|
|
|
struct drm_writeback_connector {
|
|
struct drm_connector base;
|
|
|
|
/**
|
|
* @encoder: Internal encoder used by the connector to fulfill
|
|
* the DRM framework requirements. The users of the
|
|
* @drm_writeback_connector control the behaviour of the @encoder
|
|
* by passing the @enc_funcs parameter to drm_writeback_connector_init()
|
|
* function.
|
|
*/
|
|
struct drm_encoder encoder;
|
|
|
|
/**
|
|
* @pixel_formats_blob_ptr:
|
|
*
|
|
* DRM blob property data for the pixel formats list on writeback
|
|
* connectors
|
|
* See also drm_writeback_connector_init()
|
|
*/
|
|
struct drm_property_blob *pixel_formats_blob_ptr;
|
|
|
|
/** @job_lock: Protects job_queue */
|
|
spinlock_t job_lock;
|
|
|
|
/**
|
|
* @job_queue:
|
|
*
|
|
* Holds a list of a connector's writeback jobs; the last item is the
|
|
* most recent. The first item may be either waiting for the hardware
|
|
* to begin writing, or currently being written.
|
|
*
|
|
* See also: drm_writeback_queue_job() and
|
|
* drm_writeback_signal_completion()
|
|
*/
|
|
struct list_head job_queue;
|
|
};
|
|
|
|
struct drm_writeback_job {
|
|
/**
|
|
* @cleanup_work:
|
|
*
|
|
* Used to allow drm_writeback_signal_completion to defer dropping the
|
|
* framebuffer reference to a workqueue
|
|
*/
|
|
struct work_struct cleanup_work;
|
|
|
|
/**
|
|
* @list_entry:
|
|
*
|
|
* List item for the writeback connector's @job_queue
|
|
*/
|
|
struct list_head list_entry;
|
|
|
|
/**
|
|
* @fb:
|
|
*
|
|
* Framebuffer to be written to by the writeback connector. Do not set
|
|
* directly, use drm_atomic_set_writeback_fb_for_connector()
|
|
*/
|
|
struct drm_framebuffer *fb;
|
|
};
|
|
|
|
int drm_writeback_connector_init(struct drm_device *dev,
|
|
struct drm_writeback_connector *wb_connector,
|
|
const struct drm_connector_funcs *con_funcs,
|
|
const struct drm_encoder_helper_funcs *enc_helper_funcs,
|
|
const u32 *formats, int n_formats);
|
|
|
|
void drm_writeback_queue_job(struct drm_writeback_connector *wb_connector,
|
|
struct drm_writeback_job *job);
|
|
|
|
void drm_writeback_cleanup_job(struct drm_writeback_job *job);
|
|
void drm_writeback_signal_completion(struct drm_writeback_connector *wb_connector);
|
|
#endif
|